Blog to understand automation concepts in QTP, Selenium Webdriver and Manual Testing concepts
How to send e-mail using outlook object in QTP
Labels:
QTP,
qtp Scripts,
QTP Tutorials,
Test Scripts
'' create an object of outlook application and creating a new e-mail object
'' Provide details of the mail object
'' attach a file with the e-mail, provide path of the attachment in the folder
''release the object created Set objMail = Nothing
Tutorial about Array in VBScript for QTP
Labels:
Test Scripts,
VBScript,
VBScript Tutorials
Array is a data type used to describe a collection of elements. An array can be one-dimensional or multidimensional array. In VBScript, array can be represented as follows:
Dim arrayOned(6) - represent a dimension array which can store 7 elements. Index starts
from 0.
Dim arraytwod(6)(4) - represents a two dimensional array
which can store 7*5 =35 element
Dim(6)(4)(5) - Represents a three dimensional array. We can describe
n-dimension array in similar ways.
The above arrays are example of fixed array. Once we specify
an array with dim and providing size of array, it is created as fixed dimension
array. We cannot change the size of the array.
We can also create dynamic array in VBScript as shown below:
dim arraydynam() - create a variable without providing the size of array.Redim arraydynam(10) - we can then assign a size to the array by using redim. We can resize the array size as when required.
On reducing
the size of the array, loss of information can take place, so care should be
taken while reducing the size of array in dynamic array.
There are some useful inbuilt functions in VBScript used to work with arrays. Let us discuss on the same.
Split Function - Split can be used to split a string into array based on the delimiter provided. Syntax for Split function is:
Split (expression [, delimiter [, count [, compare]]]) -
here expression denotes the string which will be splitted based on the
delimiter provided.
Count and compare are optional with default value as -1
(repeats all substring) and binary compare. Default delimiter used is space.
Ubound function - Ubound returns largest subscript for the provided dimension of an array. In Case of argument for dimension not provided, it gives the ubound for first dimension
UBound(array, dimension))
Lbound function - Lbound returns smallest subscript for the provided dimension of an array. In Case of argument for dimension not provided, it gives the lbound for first dimension
UBound(array, dimension))
Join Function - Join function does opposite of what Split function does. It joins all the elements in the array using the delimiter. Syntax of function is:Join(array, delimiter)
In case of join also, the default delimiter if not provided is
space character.
Filter Function - Filter is used to create a sub array from
array matching the particular condition.
Filter(array, value, include, compare) - In the function,
include and compare are optional parameters, include can have value true or false,
true for searching for element with particular value matching and false to
return elements which do not have match the condition
Array function - This function creates an array based on array element provided in the argument.
e.g. : a = array ("testing ", "array",
"by" ,"creating", "array", "using array
functions)
IsArray function returns true/false that indicates whether a specified variable is an array or not
''A simple code explaining the various functions for array manipulation
dim arr, strjoin
arr = split("this,is, wonderfrul, test",",") ' create an array using split function
ilowbound = lbound(arr) 'get lowerbound and upperbound for array
iupperbnd = ubound(arr)
for i=ilowboud to iupperbnd Step 1 '' Loop the array element
msgbox arr(i)
Next
b= filter(arr, "t")
msgbox b(1)
strjoin = join(arr, ";")
msgbox strjoin
msgbox Isarray(arr)
Understanding operators and built-in String functions in VBScript
Labels:
VBScript,
VBScript Tutorials
In the previous article we discuss on defining a variable, different types of variables in VBscript . In this article we will discuss on various operators and built in string functions in VBScript as are frequently used in QTP.
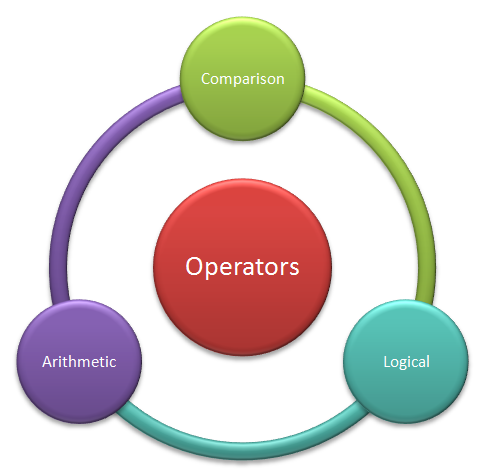
Let us start by discussing different types of operators. Operators are broadly classified into
- Logical Operators
- Arithmetic operators
- Comparison Operators
Logical Operators - Logical Operator are used to perform logical operations and returns boolean value as true or false based on the inputs provided and operator used.
Logical Operator used are Not ( Not True = false)
And (Returns true if both A and B are true, false otherwise)
OR(Returns true if either of A or B is true)
xor ( returns false if either of A or B is true)
Arithmetic Operators - We have been performing arithmetic operation from the day we start our education. So this is easy. The various arithmetic operators in VBScript are +(addition), - (subtraction), *(multiply), /(division),
\(integer division, return the quotient),
mod (a mod b, gives the remainder on dividing a by b),
^(exponential function).
Of the Operators , defined above , + is used to concatenate two strings also.
Comparison Operators are used to compare two variable . Example of comparison Operators are =(equality), <>(Inequality), <(Less than ), <(greater than), <=,>= ( greater than or equal to). To Compare strings, we can use strcomp function.
We have discussed the various operators used in VBScript.Now let us discuss on various string functions that are inbuilt in vbscript and are useful to work with strings
Instr function - This function returns the location of first occurrence of one string within another string.
str1 = "This is testing blog on VBScript testing"
str2 = "testing"
iLoc = Instr(1,str1,str2,0)
msgbox iLoc
Syntax is InStr([start,]string1,string2[,compare]). start and compare are optional parameters with default value as 1 and 0(binary compare)
Instrrev function - This function returns the location of first occurrence of one string within another string. While Instr starts search from start of string,In Instrrev The search begins from the end of string. But the location returned is from start of string.
str1 = "This is testing blog on VBSCRIPT testing test"
str2 = "testing"
iLoc = Instrrev(str1,str2,-1,0)
msgbox iLoc
Syntax is InStrRev(string1,string2,[start,][compare]). start and compare are optional parameters with default value as -1(end of string) and 0(binary compare)
LCase(string) converts string to lowercase
Ucase(string) converts to uppercase.
len(string) returns number of character in a string.
Left, right and mid are used to return specified characters from the string starting from left,right, and middle of string
str3 = left(str1,10)
str4= right(str1, 10)
str5 = mid(str1,5,10)
LTrim,RTrim and trim are used to remove spaces on the left, right or middle of a string
str6 = trim(str1)
strComp compares two string and returns value as 0 if both string matches.
blncomp = strcomp(str1,str2[,compare])
space(number) returns the space character returned times as provided in the argument.
String(number, character) returns a string with character repeated number of times as provided in the arguments.
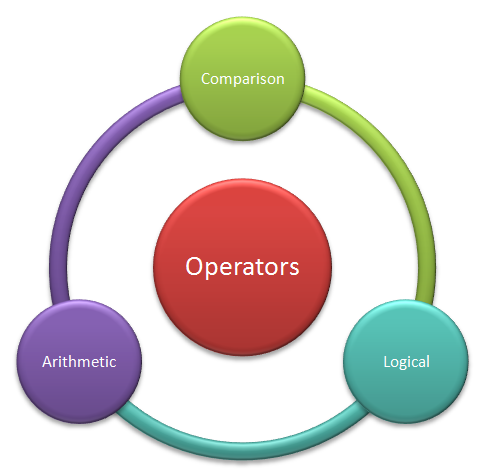
Let us start by discussing different types of operators. Operators are broadly classified into
- Logical Operators
- Arithmetic operators
- Comparison Operators
Logical Operators - Logical Operator are used to perform logical operations and returns boolean value as true or false based on the inputs provided and operator used.
Logical Operator used are Not ( Not True = false)
And (Returns true if both A and B are true, false otherwise)
OR(Returns true if either of A or B is true)
xor ( returns false if either of A or B is true)
Arithmetic Operators - We have been performing arithmetic operation from the day we start our education. So this is easy. The various arithmetic operators in VBScript are +(addition), - (subtraction), *(multiply), /(division),
\(integer division, return the quotient),
mod (a mod b, gives the remainder on dividing a by b),
^(exponential function).
Of the Operators , defined above , + is used to concatenate two strings also.
Comparison Operators are used to compare two variable . Example of comparison Operators are =(equality), <>(Inequality), <(Less than ), <(greater than), <=,>= ( greater than or equal to). To Compare strings, we can use strcomp function.
We have discussed the various operators used in VBScript.Now let us discuss on various string functions that are inbuilt in vbscript and are useful to work with strings
Instr function - This function returns the location of first occurrence of one string within another string.
str1 = "This is testing blog on VBScript testing"
str2 = "testing"
iLoc = Instr(1,str1,str2,0)
msgbox iLoc
Syntax is InStr([start,]string1,string2[,compare]). start and compare are optional parameters with default value as 1 and 0(binary compare)
Instrrev function - This function returns the location of first occurrence of one string within another string. While Instr starts search from start of string,In Instrrev The search begins from the end of string. But the location returned is from start of string.
str1 = "This is testing blog on VBSCRIPT testing test"
str2 = "testing"
iLoc = Instrrev(str1,str2,-1,0)
msgbox iLoc
Syntax is InStrRev(string1,string2,[start,][compare]). start and compare are optional parameters with default value as -1(end of string) and 0(binary compare)
LCase(string) converts string to lowercase
Ucase(string) converts to uppercase.
len(string) returns number of character in a string.
Left, right and mid are used to return specified characters from the string starting from left,right, and middle of string
str3 = left(str1,10)
str4= right(str1, 10)
str5 = mid(str1,5,10)
LTrim,RTrim and trim are used to remove spaces on the left, right or middle of a string
str6 = trim(str1)
strComp compares two string and returns value as 0 if both string matches.
blncomp = strcomp(str1,str2[,compare])
space(number) returns the space character returned times as provided in the argument.
String(number, character) returns a string with character repeated number of times as provided in the arguments.
VB Script tutorial - Variables, Constants and Arrays
Labels:
Test Scripts,
VBScript,
VBScript Tutorials
VBScript is used extensively with QTP for creating test script. With articles on VBScript, I will try to explain the VBscript and refresh the concepts for my self knowledge.
In VBscript, variables of all the data types are declared with the same data type called as variant
A variable can be defined as Dim abc. We may or may not need to define the variable explicitly. If we require every variable used must be defined. we need to provide option explicit.
Using Option explicit is a good practice as it keeps a check on the variables used in the script.
'' For e.g. in below example we define the fixed size array, on trying on resize the array, it gives us error.
dim arra(5)
An array can be defined as dynamic array, we can resize the array then, below code explains how to create a dynamic array. Preserve is used to preserve value
dim arrad() '' define a dynamic array variable without size provided
An variable defined at a function level can be used within the function in which it is defined. A variable defined at script level, can be used across the functions within the script.
const abcd = 3
Option explicit
dim abc
abc = 3
msgbox abc
Dim [1Test 231]
[1Test 231] = "I have defined a variable not starting with alphabet and having a space in between"
msgbox [1Test 231]
An array can be a fixed size array defined as dim arra(5) , this will store 6 elements, but once defined the array size can not be modified.
redim arra(3)
arra(0) = 54
msgbox arra(0)
redim arrad(3) '' Using Redim, we can define the size of the array.
arrad(3) = 75
redim preserve arrad(5) '' we use preserve the values stored on existing array using preserve as shown here. In this example we resized the array size to 5 and preserved the original value stored in array.
msgbox arrad(3)
We can also create multiple dimension array as dim arram(4)(5)(6).
Another type of variable in constant. A constant variable does not change its value once defined. Also value cannot to assigned to a constant post declaration of constant and its value
abcd = 43
msgbox abcd
In VBscript, variables of all the data types are declared with the same data type called as variant
A variable can be defined as Dim abc. We may or may not need to define the variable explicitly. If we require every variable used must be defined. we need to provide option explicit.
Using Option explicit is a good practice as it keeps a check on the variables used in the script.
Some Important point to note about variable declaration are :
- Variable for all the data type are defined as dim in vbscript
- VBScript is not case sensitive, therefore variable abc and ABC are the same.
- If Option explicit statement is defined, each of the variables used in the vbs file must be declared before use.
- A variable must start with alphabet and should not exceed 255 characters
- We can define variable everywhere in the script but it is best to define all the variable at the start of the function or script for better maintenance of variables
- We can ignore standard naming convention by defining the variable within square bracket but should avoid this.
- Multiple variable can be defined as Dim abc,def,qrtin
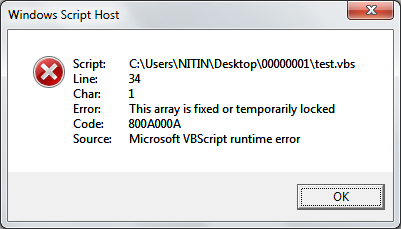
dim arra(5)
An array can be defined as dynamic array, we can resize the array then, below code explains how to create a dynamic array. Preserve is used to preserve value
dim arrad() '' define a dynamic array variable without size provided
An variable defined at a function level can be used within the function in which it is defined. A variable defined at script level, can be used across the functions within the script.
const abcd = 3
Option explicit
dim abc
abc = 3
msgbox abc
Dim [1Test 231]
[1Test 231] = "I have defined a variable not starting with alphabet and having a space in between"
msgbox [1Test 231]
An array can be a fixed size array defined as dim arra(5) , this will store 6 elements, but once defined the array size can not be modified.
redim arra(3)
arra(0) = 54
msgbox arra(0)
redim arrad(3) '' Using Redim, we can define the size of the array.
arrad(3) = 75
redim preserve arrad(5) '' we use preserve the values stored on existing array using preserve as shown here. In this example we resized the array size to 5 and preserved the original value stored in array.
msgbox arrad(3)
We can also create multiple dimension array as dim arram(4)(5)(6).
In QTP, we can also define environment variable to be used across the actions.
Another type of variable in constant. A constant variable does not change its value once defined. Also value cannot to assigned to a constant post declaration of constant and its value
abcd = 43
msgbox abcd
Subscribe to:
Posts (Atom)